I have a simple .cpp
file that depends on jsoncpp
. As a part of my build process I want Scons to untar jsoncpp (if it isn't already) and build it (if it isn't already) before attempting to compile app.cpp
since app.cpp
depends on some .h
files that are zipped up inside of jsoncpp.tar.gz
.
This is what I've tried so far:
However, Scons never prints 'Extracted jsoncpp'.. it always attempts to compile app.cpp and then promptly fails.
If I were using make
, I could simply do something like:
And the order would be guaranteed.
You should take a look at the UnTarBuilder, as a means to extract a tarfile and have all of the extracted files be properly inserted into the dependency tree. But the following will get what you have working.
You want to avoid explicit dependencies, if possible. One of the many joys of SCons is letting it take care of your dependencies for you. So just list the source file you are depending on as one of the targets of your untar command builder.
To test this I created a tar file called jsoncpp.tar.gz
containing just one file, app.cpp
, with the following contents.
SCons is a build system. It takes a bunch of input files and run tools on them to produce output. SCons is written in pure Python, works the same way on Linux, Windows and OS X, and may be run without installation. SCons' SConstruct files are Python scripts with built-in commands that create a build tree. SCons - a software construction tool Welcome to the SCons development tree. The real purpose of this tree is to package SCons for production distribution in a variety of formats, not just to hack SCons code.
And updated your SConstruct to the following.
'scons' is not recognized as an internal or external command, operable program or batch file. Because of that, I tried editing the user and system path variable to include scons under the directory 'C: Users dwayn AppData Local Programs Python38 Lib site-packages scons ', but I get the same error. Install python, including pip and tick 'add python to PATH'. Run pip install scons in command line. This installs Scons, but it won't be available from command line yet. If you run pip show scons it'll show you where the Scons code actually is (for me it's c:usersusernameappdataroamingpythonpython38site-packages - but this is not what.
Text editor for java mac. Because you list the required source file you depend on as a target of your command builder, it will handle the dependencies for you.
And when you run, you will see the following.
The reason why your code does not work is because you are listing jsoncpp
as the target of your untar command builder. Which is not a file that compiling app.cpp
will depend on, even if you list that action as an explicit dependency.
While this doesn't exactly answer your question, I hope it provides a solution to what you are trying to accomplish.
Multiple definition of class methods when building shared library
c++,linker,scons
Turns out the issue was with SConstruct. I collect my source files using the Glob command, like so: source_files = Glob('build/*.cpp') source_files = source_files + Glob('build/exceptions/*.cpp') source_files = source_files + Glob('build/gui/*.cpp') source_files = source_files + Glob('build/gui/cef/*.cpp') source_files = source_files + Glob('build/models/*.cpp') source_files = source_files + Glob('build/*.cpp') source_files = source_files +..
Override an SCons builder
I don't know if there is a blessed way to replace a Builder, but I think you're on the right track. The following (admittedly trivial) example works for me: def wrap_builder(bld): return Builder(action = [bld.action, 'echo $TARGET'], suffix = bld.suffix, src_suffix = bld.src_suffix) obj_bld = env['BUILDERS']['Object'] env['BUILDERS']['Object'] = wrap_builder(obj_bld) env.Program('test',..
Combination of chdir=1 and num_jobs>1 in SCons
python,build,multiprocessing,scons
From the SCons man page http://www.scons.org/doc/2.3.2/HTML/scons-man.html WARNING: Python only keeps one current directory location for all of the threads. This means that use of the chdir argument will not work with the SCons -j option, because individual worker threads spawned by SCons interfere with each other when they start changing..
What does “Error 309” mean?
python,c++,windows,scons,visual-leak-detector
It turns out that the magic number 309 is more googleable when written as: 0xC0000135 (no idea why C, but 135HEX 309DEC), and it is an identifier of the STATUS_DLL_NOT_FOUND error. So, it's not a SCons error, but Windows error, that leaks through SCons. This means that some DLLs..
Ignoring specific scons warnings
If you take a short look at the MAN page ( http://scons.org/doc/production/HTML/scons-man.html ), you'll find the 'warn=no-all' option..amongst a lot of other useful stuff. Note however, that switching off this warning is a bad idea in general, because it's hinting at flaws in your build description. You're telling SCons to..
Scons - Stop cleaning .obj files generated, on cleaning static library
How about: env = Environment() sources = ['tridip.c', 'tridip1.c', 'tridip2.c'] objects = [ env.StaticObject(sf) for sf in sources ] env.NoClean(objects) lib = env.StaticLibrary('tridip', objecst) exe = env.Program('tridip3.c', LIBS=lib, LIBPATH='.') ..
Config Libs produced by scons construction tool introducing LNK2019
c++,scons,lnk2019,libs
The question indeed arises from the setting of custom vs proj settings, there are three cases in which the config will pass the linker: 1: In Release Mode, MT specified 2: In Release Mode, MTd specified, add 'ITERATOR_DEBUG_LEVEL=0' in preprocessor definiton. 3: In Debug Mode, MTd specified, add 'ITERATOR_DEBUG_LEVEL=0' in..
Scons Visualstudio, multiple build targets generates duplicate NMakeOutput tag
c++,visual-studio,scons
It's a problem with your SCons/Builder syntax. You want to specify the 'runfile' parameter as well, for your two separate variants 'Debug' and 'Release'. See also the description of the MSVSProject Builder in the Appendix B of the UserGuide http://www.scons.org/doc/production/HTML/scons-user.html .
SCons Action Verbosity Levels
shell,scons,verbosity
You normally don't need to use Execute() in a builder action. Perhaps if you share that bit of code it might help. Microsoft exchange outlook for mac. You could also look into using a generator, depending on what exactly you're looking for.
scons not using PATH for ParseConfig
scons,sdl-2
Yes. SCons does not use the user's path by default. You need to pass the PATH explicitly to the Environment: import os env = Environment(ENV = {'PATH' : os.environ['PATH']}) See the SCons FAQ..
How to tell scons to exclude certain files from using the build cache
caching,scons
There is NoCache: P = Program(..) NoCache(P) ..
Overriding SCons Cache Copy Function
python,caching,scons,build-system,hardlink

Sconce
We finally figured out that import SCons.Environment SCons.Environment.Environment._copy_from_cache = link_or_copy_file SCons.Environment.Environment._copy2_from_cache = link_or_copy_file worked..
CCCOMSTR/LINKCOMSTR for SharedLibrary in SConscript will not work
c,scons
After some research I found the answer and now it displays it properly: .. env['SHCCCOMSTR'] = 'SHCC $SOURCE' env['SHLINKCOMSTR'] = 'SHCC $TARGET' env['CCCOMSTR'] = 'CC $SOURCE' env['LINKCOMSTR'] = 'LINK $TARGET' .. I thought I had tried this before and it didn't work, but obviously something was wrong since it works..
scons error using Glob in SConscript
Your problem is that you're using variant_dir to 'link' your build folder to '.' as your source directory. Combined with the default 'duplicate=1' option, this means that SCons tries to replicate all sources into 'build'..but the latter is part of the source folder too. This opens the door to all..
Scons and external directories
python,scons
You should consider using the SCons Install Builder. This is the only way to install targets outside of the SCons project hierarchy.
scons - always install after build
I went the wrong way of defining install target and trying to figure out how to run it automatically. I solved it by aliasing the Install builder to the real file names I wanted to install instead of install. Env.Alias('/some/dir/filename', Env.Install('/some/dir', Target)) ..
How to stop Scons adding lib infront of a shared library
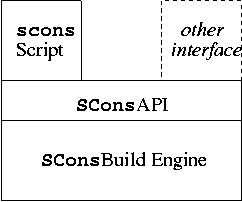
c++,osx,dll,scons,dylib
In each environment, SCons uses variables to specify the prefixes and suffixes of things like libraries and programs. These variables get initialized, based on the detected platform that it's currently running on..but you can simply overwrite this setting after the call of the Environment() constructor: env = Environment() env['SHLIBPREFIX'] =..
Running an ANT script from SCONS
java,android,ant,scons
It depends on how and when you want to execute ant, but yes it is indeed possible. If you want to execute this ant script every time SCons executes, then you can use the SCons Execute() function, as follows: env.Execute('/usr/local/bin/ant release -f /Users/dev/pic/src/platform/android/java/build.xml') This will execute every time, with no..
Scons - How to stop cleaning dependent targets/files on cleaning final target?
You need the NoClean function. Here is how to update your SConstruct. env = Environment() src1 = ['tridip.c', 'tridip1.c', 'tridip2.c'] obj1 = [ env.StaticObject(sf) for sf in src1 ] lib = env.Library('tridip', obj1) if 'library' not in COMMAND_LINE_TARGETS: env.NoClean([obj1, lib]) Alias('library', lib) src2 = ['tridip3.c'] obj2 = [ env.StaticObject(sf) for..
How to create dynamic defines for Visual Studio?
c++,visual-studio,visual-studio-2013,define,scons
A common way to do this is to define your constants in an include file: e.g. // Version.h - Autogenerated, don't edit #define VERSION_MAJOR 1 Next you write a script or a program (in your favourite language) to obtain version from somewhere and dynamically write Version.h. Possibly parse the old..
Faulty D Dependency Logic in SCons
python,regex,import,d,scons
Short Answer To handle all the cases you have outlined, try the following twist on your changes to the (self.cre) pattern: imports+(?:[a-zA-Z0-9_.]+)s*(?:(?:s+:s+[a-zA-Z0-9_.]+s*)?(?:,s*(?:[a-zA-Z0-9_.]+)(?:s*:s*[a-zA-Z0-9_.]+)??s*)*)*; Debuggex Demo Digging Deeper self.cre vs. self.cre2 Yes, the find_include_names method.. def find_include_names(self, node): includes = [] for i in self.cre.findall(node.get_text_contents()): includes = includes + self.cre2.findall(i) return includes..
Does anything happen at build time that is specific to static linking
c++,linker,static-linking,scons,dynamic-linking
In the general case you can't use static and dynamic libraries interchangeably. See: Static link of shared library function in gcc Regarding your actual question: .. if there is any difference between .o files that were destined to be statically linked to the libraries and .o files that were destined..
How to debug parallel scons builts
I recently had similar problems in my build system at work. The first problem I encountered was very strange, as it seemed like SCons was completely messing up the directory it was supposed to be in. Upon consulting the SCons user's mailing list, I was told that you cant change..
scons and ObjC++
objective-c,osx,scons
I checked the sources of the current version 2.3.4 and there is no support for the FRAMEWORKS variable when compiling source files, only for linking (applelink.py Tool). So you'd have to define your own ObjC Builder, which then could use the already defined variables like $_FRAMEWORKPATH, $_FRAMEWORKS and $FRAMEWORKSFLAGS. If..
Scons: What is the purpose of `FORTRAN*` variables when building Fortran?
fortran,scons
Looking through the SCons source (particularly Tool/FortranCommon.py) it appears the that FORTRAN is treated as a dialect along with F77, F90, F95 and F03 rather than a parent to all of them. It looks like the FORTRAN variant of the variables will be used for source files named with .f,..
scons - delete some of the built files after the compilation process
python,c++,swig,scons
It seems like using the 'Delete' function as a Command, after the SharedLibrary line, allows me to delete the unwanted files.
Why is SCons saying that my hpp file is static and cannot be used to make a shared library?
shared-libraries,scons
Just don't add the header to the list of direct sources for the lib. Set the CPPPATH variable correctly and let SCons find the header itself, which will add it as implicit dependency. For more infos on this, check out the SCons UserGuide at http://www.scons.org/doc/production/HTML/scons-user.html , '6.3 Implicit dependencies'. (Remark:..
Using scons to compile c++ file with -std=c++11 flag
c++11,scons
Use env.Library instead of Library to build the library with your construction environment. env = Environment() env.Append(CXXFLAGS = '-std=c++11') src_files = Split('test.cc') lib_name = 'test' env.Library(lib_name, src_files) ..
Additional, specific source and target for a Builder
From what you describe, you should be using both an emitter and a generator, just as you state at the end of your question. The 'main' source/target will be the first element in the source/target lists. This doesn't seem hacky to me, but I may just be used to it...
scons fails to notice errors in commands with pipes ('|')
Sconset
pipe,scons
Your assumption is wrong: it's not SCons that thinks the command was successful. On my Linux system the command > cat test.txt | fribblecat | cat > out.txt yields 'fribblecat: command not found.' and an immediate > echo $? gives a '0' (success) as result. So the shell opens the..
Adding C# support to SCons on Mac
c#,python,scons
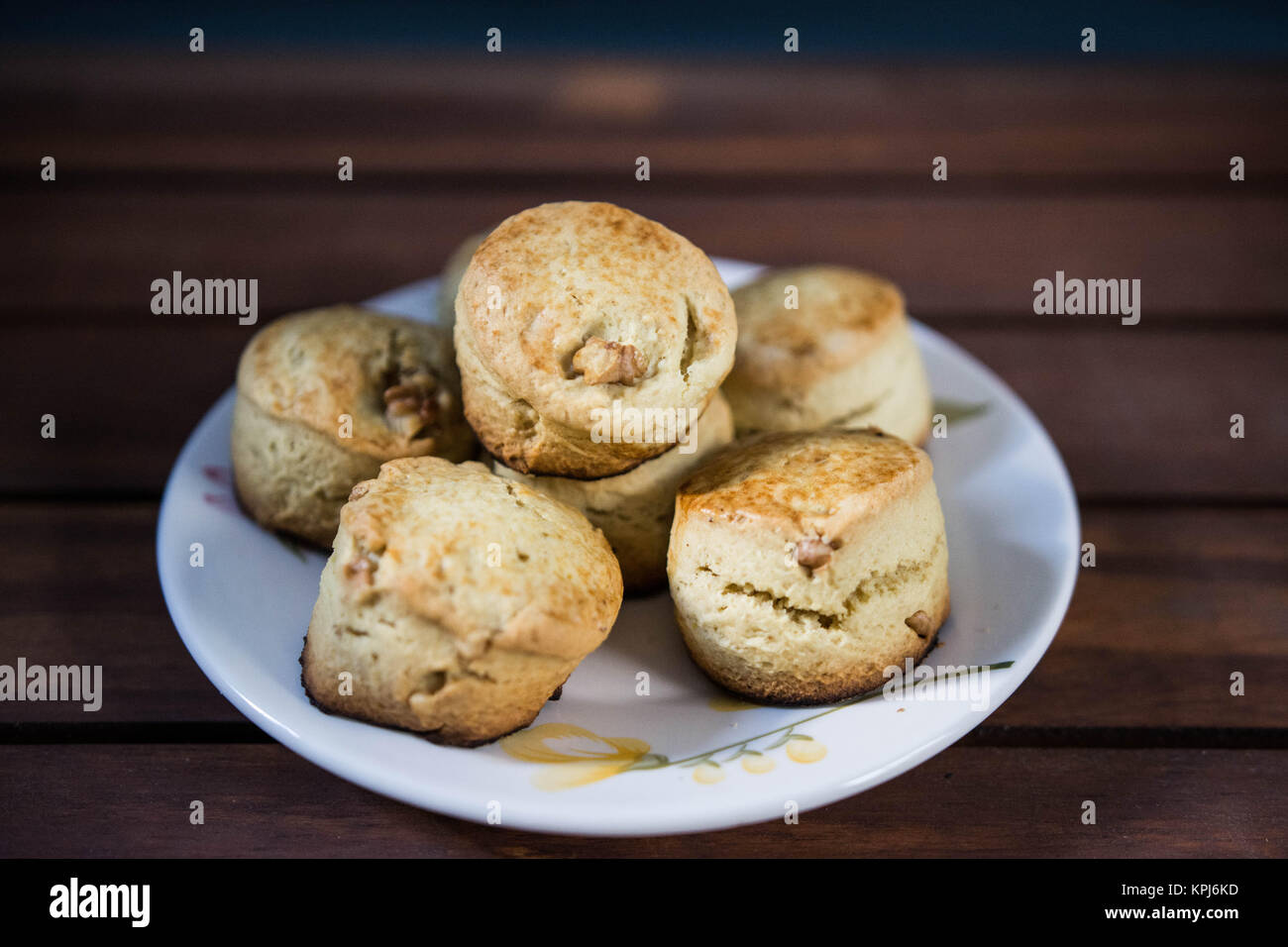
Create a directory ~/.scons/site_scons/site_tools. cd ~/.scons/site_scons/site_tools hg clone https://bitbucket.org/russel/scons_csharp Change one line (460) from csharp.py (~/.scons/site_scons/site_tools/scons_csharp/csharp.py). env['CSC'] = env.Detect('mcs') or 'csc' We need this change because the default setting for compiler (gmcs) is outdated. Create build file: SConstruct. env = Environment( tools=['scons_csharp'] ) sources = ['Hello.cs'] prog = env.CLIProgram('myapp',..
How to export a program with an alternate extension in SCons on Windows
compilation,compiler-errors,cross-compiling,scons
The call prog = env.Program('progname.elf', obj, libs) in your SConscripts should look like this: prog = env.Program('progname', obj, libs) . Then you could set the variable '$PROGSUFFIX' to '.elf' under Posix systems. This would be the SConsish way of dealing with this..see also How to force scons to generate binary..
Wrap a scons build process in a Makefile
makefile,scons
Scon Stock Price Target
After looking for such a solution some time ago, I ended up writing a Makefile for this purpose. Because SCons also comes as a drop-in userspace package scons-local (see the download page), one can fetch in and run it. Here is a dissectioned and commented version of my Makefile, which..
How can I set the TARGET.dir property in Scons?
python,scons
The 'TARGET' in the Command refers to the path of the first given target, and 'TARGET.dir' returns its folder (=dirname) dynamically. So you can't easily change the output dir without touching the SConstruct..
Scons Explicit Dependency
You should take a look at the UnTarBuilder, as a means to extract a tarfile and have all of the extracted files be properly inserted into the dependency tree. But the following will get what you have working. You want to avoid explicit dependencies, if possible. One of the many..
How to add extra clean targets while using SCons
One way to do this is to create you're own builder, then modify the emitter() function to include those possible extra targets. In my own progject, I created my own builder around the SWIG tool because when it executes, I was saving the generated .py file in a different directory..
